Variables : All About Java Variables
In this Article, we will learn what are Variables in java and how to use them with their syntax and some real life examples.
- Last Updated : July 23, 2023
- Read Time: 2 Minutes
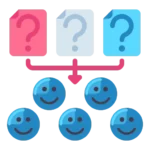
Article’s Content
What are Variables?
Variables are used to store and manipulate data in programs. Each variable has a specific data type, which determines the kind of values it can hold.
Declaration:
Before using a variable, you need to declare it, specifying its name and type.
int age;
Initialization:
Variables can be initialized with an initial value at the time of declaration or later in the program.
int age = 25;
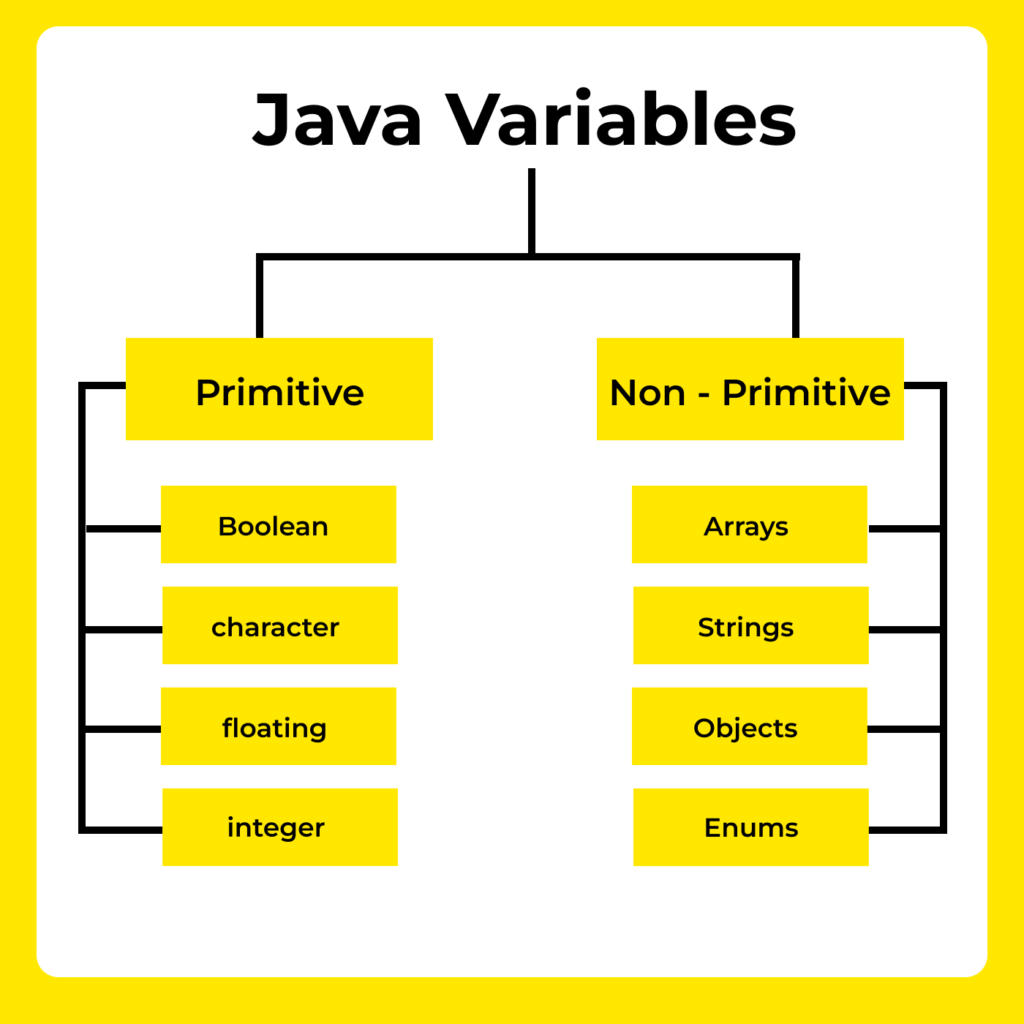
Types of Variables:
Variables in Java have types that define the kind of data they can hold. Some common types include:
int
(integer)double
(floating-point number)boolean
(true/false)char
(character)String
(sequence of characters).
Scope of Variables:
Variables have a scope, which defines where they can be accessed in the program. Local variables are declared inside methods, constructors, or blocks and can only be used within that specific context. Instance variables are declared within a class but outside any method and can be accessed by all methods of the class. Class variables, also known as static variables, are shared by all instances of a class.
Description:
In Java, variables are used to store and manipulate data during the execution of a program. They act as containers that hold different types of values, such as numbers, text, characters, and more. Variables make it possible for the program to remember and use data at different points in the code.
Here’s a step-by-step explanation of variables using a real-time example:
Example : Online Shopping Cart :
Imagine you are building an online shopping cart application. Users can add items to their cart and then proceed to checkout.
Step 1 : Declaring Variables
To represent the shopping cart, you’ll need a few variables. In this case, you can use arrays and primitive data types.
// Declare variables to hold item details String[] cartItems = new String[5]; // An array to store item names (up to 5 items) double[] itemPrices = new double[5]; // An array to store item prices (up to 5 items) int itemCount = 0; // A variable to keep track of the number of items in the cart double totalAmount = 0.0; // A variable to store the total amount of the cart
Step 2: Adding Items to the Cart
Let’s assume the user adds two items to the cart: a “T-shirt” for $20 and “Shoes” for $50.
// Add the first item to the cart cartItems[itemCount] = "T-shirt"; itemPrices[itemCount] = 20.0; totalAmount += itemPrices[itemCount]; itemCount++; // Increment the itemCount to 1 // Add the second item to the cart cartItems[itemCount] = "Shoes"; itemPrices[itemCount] = 50.0; totalAmount += itemPrices[itemCount]; itemCount++; // Increment the itemCount to 2
Step 3: Displaying Cart Information
After adding items to the cart, you can display the cart details to the user.
System.out.println("Items in the cart:");
for (int i = 0; i < itemCount; i++) {
System.out.println(cartItems[i] + ": $" + itemPrices[i]);
}
System.out.println("Total amount: $" + totalAmount);
Output :
Items in the cart:
T-shirt: $20.0
Shoes: $50.0
Total amount: $70.0
Explanation:
In this example, we use variables like cartItems, itemPrices, itemCount, and totalAmount to represent the shopping cart’s contents and information. The itemCount variable helps keep track of how many items are in the cart, and the totalAmount variable is used to calculate and store the total cost of the cart.
These variables allow us to dynamically store and manage data related to the shopping cart, providing a realistic demonstration of how variables are used in Java for real-time applications.
Java Program to Demonstrate Variables in Java:
// Java Program to demonstrate // Instance Variables import java.io.*; class GFG { // Declared Instance Variable public String geek; public int i; public Integer I; public GFG() { // Default Constructor // initializing Instance Variable this.geek = "Shubham Jain"; } // Main Method public static void main(String[] args) { // Object Creation GFG name = new GFG(); // Displaying O/P System.out.println("Geek name is: " + name.geek); System.out.println("Default value for int is " + name.i); // toString() called internally System.out.println("Default value for Integer is " + name.I); } }
Author : Lakshit Mittal
Revised By :